|
2022-2023 Catalog [ARCHIVED CATALOG]
Course Descriptions
|
|
Key to Course Descriptions
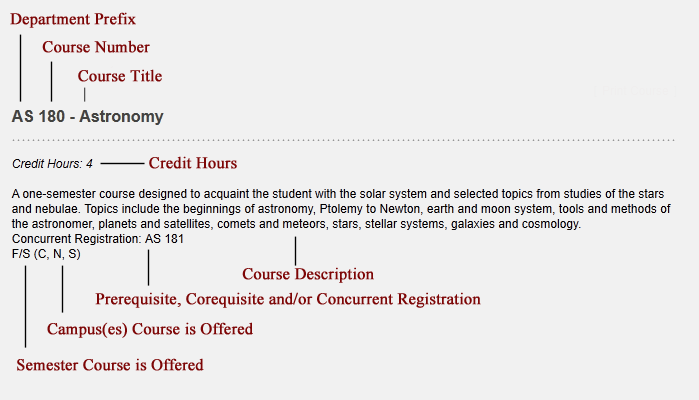
Abbreviations
(C) |
City Campus |
F/S |
Fall and Spring |
(N) |
North Campus |
SS |
Summer Session |
(S) |
South Campus |
F+ |
Offered every other Fall |
F |
Fall |
S+ |
Offered every other Spring |
S |
Spring |
N |
Non-Credit |
Course Outlines
Course outlines for all courses described in this catalog are available for viewing. To explore the general framework of a course design and view the expectations of student performance within a select course click on the link below. These descriptions provide the base upon which instructors build their own course syllabi for the individual sections offered by the academic departments. Individual sections may therefore vary somewhat from the descriptions given in the outlines.
Course Outlines
|
|
Computer Science |
|
-
CS 121 - Computer Science I Credit Hours: 4
An introductory level survey course in computer science which will include the following topics: basic components and organization of a computer, machine representation of data, number systems, nature and design of algorithms, top down development of programs, data types, control structures and basic data structures. A structured programming language (such as C++) will be studied and utilized along with operating system and editor commands. Students will be required to complete several programming projects.
Course Outcomes Upon completion of this course, the student will be able to:
- identify basic components and organization of a computer;
- demonstrate the use of the operating system and an editor to write and execute programs;
- develop algorithms for solving problems on a computer utilizing a top-down approach with stepwise refinement;
- write, debug and execute programs in a structured programming language;
- use basic datatypes, expressions, and built-in functions to write elementary programs;
- use user created and library functions. Differentiate between input and output (value, reference) arguments;
- differentiate between iteration and recursion, and trace functions of each type;
- implement the control structures of the language;
- write modular solutions by use of multiple files for implementation of abstract data types; and
- use user created classes.
- identify problems where the use of arrays to store data is appropriate, and define the data types using arrays;
- compare and analyze basic sorting algorithms: selection, insertion, exchange;
- compare and analyze searching techniques: linear, binary;
- write user documentation for programs explaining the purpose of the program to a user;
- write programmer documentation explaining the implementation of the program to another programmer; and
- technology objectives: Students will be able to demonstrate “hands on”proficiency in using current hardware, software tools and languages to accomplish all of the above course objectives.
Prerequisites: MT 125 or waived from placement test or placed into degree credit math F/S (N, S)
|
|
-
CS 132 - Computer Science II Credit Hours: 4
A continuation of CS 121. Further study of the organized design, development and testing of computer programs. Emphasis will be on recursion and on the design and application of the important abstract data types/structures of computer science: stacks, queues, singly and doubly linked lists and trees. Several programming projects using these data structures will be required.
Course Outcomes Upon completion of this course, the student will be able to:
- identify problems where the use of arrays to store data is appropriate, and define and use data types using arrays;
- identify problems where the use of ADT/class is appropriate;
- identify problems where the use of structures to store data is appropriate and define and use data types using structures;
- define a stack and its associated operations and implement it in a program;
- define a queue and its associated operations and implement it in a program;
- convert expressions between infix, prefix, and postfix notation;
- compare and differentiate between a recursively-defined function and an iteratively defined function;
- write, debug, and execute programs involving recursion;
- use pointers to implement new data types using dynamic storage;
- define and use linked lists, circular lists, and doubly linked lists;
- define a binary tree structure and its associated operations and implement it in a program;
- write and use generic programming from both user created templated ADT’s (classes, lists, queues, stacks, trees, etc.) and the Standard Template Library (STL);
- compare and analyze more advanced sorting algorithms: quick merge sort, heap sort, and tree sort;
- compare and analyze searching techniques: linear, binary, and tree;
- develop several implementations for a new data type, and be able to compare them in terms of space, time and ease of use;
- write careful and correct documentation for a program, explaining to a user or another programmer what the program does and how it does it; and
- technology objectives: Students will be able to demonstrate “hands on ” proficiency in using current hardware, software tools and languages to accomplish all of the above course objectives.
Prerequisites: CS 121 F/S (N)
|
|
-
CS 209 - Programming in Java Credit Hours: 4
A study of the Java programming language for students who already have programmed in another language. Major topics include object-oriented programming, class hierarchies and inheritance, encapsulation, syntax, data types/structures, control structures, graphical user interface components, exceptions, files and streams, applications and applets.
Course Outcomes Upon the completion of the course, the student will be able to:
- design, write, debug, and execute applets and programs in Java using one of the popular development environments;
- design, write, debug, and execute applets and programs in Java using an object-oriented approach;
- design, write, and debug applets in Java that run in a World Wide Web browser such as Microsoft Explorer, or Mozilla;
- use the existing standard Java class libraries and their Application Programming Interfaces (APIs) to develop applets and programs in Java;
- design, write, and debug applets and programs in Java that include use of class data members and member methods;
- use the standard Java data types (e.g., integers, double, arrays) to write applets and programs in Java;
- use the standard Java control structures to write applets and programs in Java; and
- design, write, and debug applets and programs in Java that use a multi-level class hierarchy and inheritance;
- design, write, and debug applets and programs in Java that use method and variable overriding and overloading;
- design, write, and debug applets and programs in Java that include graphics components;
- design, write, and debug applets and programs in Java that include graphical user interfaces (GUIs) and components;
- design, write, and debug applets and programs in Java that use files and streams;
- design, write, and debug applets and programs in Java that consist of applications in other disciplines such as the sciences, engineering and business;
- write documentation that is included in the internal program modules;
- TECHNOLOGY OBJECTIVES: Students will be able to demonstrate “hands on” proficiency in using state-of-the-art computer software tools and languages to accomplish the above course objectives. All of the above course objectives involve the use of computer and information technology.
Prerequisites: CS 121 or DA 140 or MT 191 or permission of the instructor. F/S (N)
|
|
-
CS 210 - Programming in C++ Credit Hours: 3
A study of the C++ programming language for students who already have programmed in another language. Major topics will include modular design, control structures, functions, data structures, pointers and the use of libraries.
Course Outcomes Upon completion of this course, the student will be able to:
- design, write, debug, and execute programs in C++ using a modular approach;
- use standard C++ data types in expressions, conversion of types, and built-in C++ functions in a program;
- use the control structures provided by the C++ language to write loops and selections;
- define and use functions with both input and output parameters. This should include recursive functions;
- define and use pointer variables;
- use arrays to store data and describe the relationship between arrays and pointers;
- define and use structures and unions to store data in a program;
- use dynamic memory allocation to create linked lists;
- use the standard C++ libraries;
- compare sequential file access and random access and use both in a program;
- define and use classes with appropriate data members and function members;
- define and use overloaded operators;
- use templates to define generic classes and generic functions;
- define and use class inheritance; and
- explain several differences between C and C++.
Prerequisites: CS 121 or DA 130 or MT 191 or permission of the instructor. F/S (N)
|
|
-
CS 211 - Computer Networks and Internetworks Credit Hours: 4
A study of how computer networks and internets operate, from the lowest level of data transmission and wiring to the highest level of application software communication over the network infrastructure. Topics will include exploration of networks and their management in the web environment, the OSI model, data and packet transmission, topologies, hardware, client-server systems, internetworks, simulation and management tools, e-commerce and security.
Course Outcomes Upon completion of this of this course, the student will be able to:
- setup a network card for use under Linux.
- setup a network printer under Windows server 2000, UNIX.
- explain the difference between baud rate and bits per second.
- design, write, debug, and execute a program to contact a web server and extract and print the returned page.
- describe how an IP router uses a routing table to forward datagrams.
- describe how a datagram crosses the Internet.
- describe the operation of error detection codes and cyclic redundancy codes.
- explain the difference between a hub and a layer 2 switch.
- describe how TCP identifies a connection.
- explain how a web server can handle multiple connections to port 80.
- explain the difference between a bridge and IP router.
- trace and compute the length of the path of a single bit as it travels across a base T network.
- explain why TCP is classified as end to end.
- explain the difference between CSMA/CD in Ethernet and a token passing scheme used in Token Ring.
- design, write, and debug socket programs for network communication between workstations and servers.
- capture and decode packet contents.
- explain the operation of public key and double key encryption schemes.
- understand the use of overflow attacks and their prevention
- describe Unix and Windows network attacks and means to defend the attacks.
- technology objectives:
- use a computer to develop programs; and
- use the Linux operating environment effectively.
Prerequisites: CS 121 or permission of the instructor. F (N)
|
|
-
CS 215 - Web Development and Programming I Credit Hours: 4
This course is the first course of a two semester sequence covering the development of Web-based software for intranets and internets. The two course sequence will cover end-to-end development, including both the client-side and server side development. Topics include design of a Web site and Web pages, Hypertext Markup Language (HTML), style sheets, scripting languages, dynamic Web pages, database connectivity, Web servers, basic server side programming and the Extensible Markup Language (XML). Modern development tools will be used.
Course Outcomes Upon completion of this course, the student will be able to:
- design and implement the folder/directory structure for a simple web site or for a new portion of an existing site;
- design and implement web pages for a web site using HTML; test and debug the pages;
- apply good design principles regarding issues such as simplicity, the use of white space, consistency across pages, visual appeal, and navigation;
- apply the design principle of separating style and structure using style sheets;
- design and implement web pages that are viewable using major web browsers;
- design and implement dynamic web pages using a scripting language with HTML; test and debug the pages;
- design and implement web pages that include basic non-text elements such as images and/or animation;
- design, implement, and test web pages that employ database connectivity;
- explain the nature and purpose of a web server and identify a few of the popular ones;
- explain the significance of the client-server software architecture for web applications;
- design, implement, and test web pages that employ XML;
- explain applications of web-network technology in industry; usage of internets and intranets in industry; and basic development practices and procedures used in industry;
- work as a member of a team to design, develop, test, and debug software for an intranet or internet web site; and
- technology objectives: Students will be able to demonstrate “hands on” proficiency in using state-of-the-art computer software tools and languages to accomplish the above 13 course outcomes. All of the above 13 course outcomes involve the use of computer and information technology.
Prerequisites: CS 121 or permission of the instructor F/S (N)
|
|
-
CS 216 - Advanced Web Development and Programming II Credit Hours: 4
This course is the second course of a two semester sequence covering the end-to-end development of Web-based software for intranets and internets. This course emphasizes server-side development of enterprise applications. Topics include Web servers, distributed network -based computing, handling client requests, server-side services, transmitting data using HTTP, database connectivity, security and e-commerce. Programming languages and tools will be among the most significant such as Java, servlets, JavaServer Pages, Active Server Pages, .NET and XML, among others.
Course Outcomes Upon completion of this course, the student will be able to:
- explain and describe distributed enterprise models and implementation alternatives;
- design and implement one or more Java servlets; test and debug the servlets; deploy the servlets;
- design and implement one or more JavaServer Pages; test and debug the JSPs; deploy the JSPs;
- design and implement server-side software that interacts with a database for the purposes of querying the database; test and debug the software; deploy the software;
- design and implement server-side software that interacts with a database for the purposes of performing insert, update, and delete operations on the database; test and debug the software; deploy the software;
- design and implement one or more Active Server Pages; test and debug the ASPs; deploy the ASPs;
- design and implement one or more COM modules; test and debug the COM modules; deploy the modules;
- design and implement an XML application that provides for information exchange; test and debug the software;
- explain important security issues and describe the technology available to address the issues;
- explain important e-commerce issues and describe the technology available to address the issues;
- explain the important ethical issues and describe the technology available to address the issues;
- identify and explain the high-priority software quality factors (e.g., scalability, performance, platform independence, reliability) and describe approaches to address these factors;
- explain applications of web-network technology in industry; usage of internets and intranets in industry; and basic development practices and procedures used in industry;
- work as a member of a team to design, develop, test, and debug software for an intranet or internet web application; and
- technology objectives: Students will be able to demonstrate “hands on” proficiency in using state-of-the-art computer software tools and languages to accomplish the above 14 course outcomes. All of the above 14 course outcomes involve the use of computer and information technology.
Prerequisites: CS 215 or permission of the instructor. S (N)
|
|
-
CS 220 - Advanced Programming in Java Credit Hours: 4
This course is a continuation of Programming in Java I (CS 209). Topics include: advanced topics in object-oriented programming, advanced graphical user interfaces (GUIs), multithreaded programs, networking, database connectivity, structured data types, server-side software including servlets and Java Server Pages, reusable integrable components and enterprise applications.
Course Outcomes Upon completion of this course, the student will be able to:
- design, write, debug, and execute Java programs using an object-oriented approach;
- use the existing standard Java class libraries and their Application Programming Interfaces (APIs) to develop Java programs;
- design, write, and debug Java programs that include the use of vectors and other structured data types;
- design, write, and debug Java programs that include the use of the capabilities of the Java Collections class;
- design, write, and debug multithreaded Java programs;
- design, write, and debug Java programs that use client-server and other technology to implement distributed systems for use on computer networks, both LANs and WANs;
- design, write, and debug Java programs that incorporate Java Database Connectivity and data retrieval from a relational database;
- design, write, and debug Java programs that include server-side software such as servlets;
- design, write, and debug Java programs that include the use of Java Server Pages (JSP);
- design, write, and debug reusable, integrable modules/components, including Enterprise JavaBeans;
- design, write, and debug enterprise applications;
- design, write, and debug Java programs that consist of applications in other disciplines such as the sciences, engineering, and business;
- write documentation that is included in the internal program modules; and
- technology objectives: Students will be able to demonstrate “hands on” proficiency in using state-of-the-art computer software tools and languages to accomplish the above course objectives. All of the above course objectives involve the use of computer and information technology.
Prerequisites: CS 209 or permission of the instructor. S (N)
|
|
-
CS 221 - Machine Organization and Assembly Language Programming Credit Hours: 4
A study of computer organization and assembly language programming. Topics include basic machine architecture and design, digital logic circuits, digital components, central processing unit, machine representation of instructions and data, addressing techniques, memory organization and execution of instructions at machine level. Assembly language programming topics include: syntax, instruction types, control structures, data types, subroutines, input/output, macros, hybrid-programs and the programming language translation process. Several programming projects will be required of each student.
Course Outcomes Upon completion of this course, the student will be able to:
- describe the general organization and main components of a computer system – the CPU, memory, peripherals, and bus;
- describe and explain the Fetch-Execute cycle of a computer;
- describe and explain the basic elements and circuit types at the digital logic level;
- describe and explain the different devices, memory components, memory types, chips, buses, as well as circuit synthesis;
- describe, explain, and use register transfer language and notation;
- compare and contrast different addressing modes;
- translate instructions into the binary form that is used for the internal representation of instructions in memory;
- translate various types of data values into the binary form that is used for the internal representation in memory;
- explain the concept of a subroutine – how control is transferred and how the arguments are passed;
- explain and describe the concept of virtual memory and the basic schemes involved in memory management;
- describe and explain the concept of an assembler and linker, as well as the assembly and link processes;
- assemble, link, and execute programs using assembly language for the Intel-86 family of microprocessors;
- design, write, execute, and debug assembly language programs;
- design, write, execute, and debug programs using subroutines;
- design, write, execute, and debug a hybrid program that has modules written in assembly language and in a high level language such as Modula, Pascal, or C;
- use the debugger to examine the actual internal representation of data and program instructions, as well as the runtime stack as a program executes;
- explain the concept of a programming macro, compare it with a subroutine, and use it in a program;
- explain the concept of conditional assembly;
- describe methods of coding data for transmission;
- write careful and correct software documentation including user and programmer manuals which explain, to a user and programmer respectively, what the program does and how it does it; and
- research a topic related to the topics in this course.
- technology objectives:
- Use computer software to create source files, assemble and/or compile files, and link files to produce an executable program;
- Use the computer network to store and retrieve files; and
- Send files in text form and binary form across the internet.
Prerequisites: CS 132 F (N)
|
|
-
CS 232 - Advanced Data Structures Credit Hours: 4
A study of advanced abstract data types used in computer science. The course will include a review of the basic data structures such as stacks, queues (including priority queues) and linked lists. Major topics will include trees, tables, hash tables, graphs, primary and secondary memory, searching and sorting. Measures of efficiency and complexity analysis will be applied throughout the course. A higher level language such as C++, including STL, will be used. Several programming projects will be required
Course Outcomes Upon completion of this course, the student will be able to:
- describe and explain: (a) the concept of an abstract data type (ADT) and (b) different algorithmic strategies;
- design, write, execute, and debug programs in C++;
- apply measures of efficiency for algorithms and ADTs and interpret the results;
- explain and describe the structure representation and access procedures for ADTs including arrays, stacks, queues, linked lists, trees, graphs, and files;
- explain and describe the applications of ADTs including arrays, stacks, queues, linked lists, trees, graphs, and files;
- explain and describe different search and internal sorting algorithms;
- explain and describe the structure, representation, and access procedures for static and dynamic tree tables and hash tables;
- explain and describe the applications of static and dynamic tree tables and hash tables;
- identify problems where advanced ADTs are appropriate and select or design the most suitable ADT for the given task;
- design, implement, and use advanced ADTs;
- identify and perform all phases of the software system development process;
- write software documentation, including user and programmer manuals; and
- technology objectives: Students will be able to demonstrate “hands on ” proficiency in using current hardware, software tools and languages to accomplish all of the above course objectives.
Prerequisites: CS 132 S (N)
|
|
-
CS 290 - Computer Science Internship Credit Hours: 4
This course is intended to provide the student with real world experience in the area of computer science. The student will be assigned a project that will entail hands-on experience in the software development process, including problem definition, requirement specifications, design, implementation, testing and debugging, and documentation. The student will typically be working with an external organization, such as a local company, to address real world problems.
The student is required to perform a minimum of 180 hours of work on the internship project as defined by the contract drawn up between student, supervisor at company, and SUNY Erie faculty member.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate ability to work in a non-academic (industry) environment;
- demonstrate ability to work as a member of a team;
- demonstrate knowledge of the software development process;
- demonstrate ability to analyze and specify requirements;
- demonstrate ability to design software and document the design with annotated design diagrams;
- demonstrate ability to implement the software design by writing the programming language code with good internal documentation;
- demonstrate ability to test and debug the software and document the final results;
- demonstrate ability to iteratively refine the software and relevant documentation;
- demonstrate ability to apply and adhere to good software design principles;
- demonstrate ability to apply and adhere to good software programming principles;
- demonstrate ability to apply and adhere to good testing and debugging principles;
- demonstrate ability to apply and adhere to good documentation principles; and
- technology objectives: Students will be able to demonstrate “hands on” proficiency in using state-of-the-art computer software tools and languages to accomplish the above course objectives. All of the above course objectives involve the use of computer and information technology.
Prerequisites: ( CS 209 and CS 215) or permission of the instructor F/S, SS (N)
|
Construction Management Engineering Technology |
|
-
CO 120 - Architectural Blueprint Reading Credit Hours: 3
A basic course in the reading of architectural blueprints for residential and commercial buildings, as well as basic sketching techniques for graphic presentation of ideas and problems.
Course Outcomes Upon successful completion of this course, the student will be able to:
- read an architect’s scale;
- identify information from multi-views and isometrics;
- explain the general kinds of information shown on various drawings used in the construction industry;
- interpret symbols and conventional representations; and
- be familiar with relevant building codes and zoning ordinances.
F/S (C, N, S)
|
|
-
CO 137 - Introduction to Welding Credit Hours: 3
This course includes topics on general welding safety, along with material cutting using Oxy-Fuel and Plasma-Arc cutting systems. Students will learn how to cut metal stock in varying thicknesses with emphasis on straight, curved and bevel cuts. Students will also learn how to use the proper protective equipment, and how to practice personal safety regarding setup, use and proper machine maintenance. Students will experience hands-on lab work.
Course Outcomes Upon completion of this course, the student will be able to:
- safely setup and use Plasma-Arc and Oxy-Fuel systems;
- present information and ideas about cutting operations effectively in various contexts and formats, both written and oral;
- properly cut and finish metal stock of varying thicknesses using straight line, curve or bevel cuts using the appropriate equipment; and
- demonstrate knowledge of welding safety including, personal safety equipment, machine safety and shop safety.
F/S (N)
|
|
-
CO 140 - Construction I Credit Hours: 3
A course covering the fundamental principles of materials, installation methods, safety and management used in residential construction. Laboratory project involves the physical application of topics discussed in lecture and computer based record-keeping of labor performances in residential construction. Course focuses on traditional or prefabricated construction methods used in producing foundation systems, sub-floor systems, wall systems, & roofing systems.
Course Outcomes Upon successful completion of this course, the student will be able to:
- identify individual building components relating to foundation systems, framing systems, exterior and interior finish systems and roofing systems for residential construction;
- basic knowledge with the use and layout of building materials in residential construction;
- basic knowledge with the use of building codes in residential construction;
- understanding how a set of residential construction drawing documents are used in the industry;
- an appropriate use of construction techniques and skills to maximize the use of materials and modern tools within the construction industry;
- an ability to apply creativity in the design of systems, components or processes appropriate to lab project objectives;
- an ability to function effectively on teams;
- an ability to identify, analyze, and solve technical problems with methods of project component installations;
- an ability to communicate effectively through project assignments and notebook submissions;
- an ability to use computers with Microsoft office products;
- use of the internet to locate materials and equipment for construction; and
- use of local network printers.
F/S (N)
|
|
-
CO 147 - Introduction to Shielded Metal Arch Welding / Stick Welding Credit Hours: 3
This course is an introductory class to shielded metal-arch welding/stick welding (SMAW). Topics covered will include: material preparation, machine setup and safety, welding terminology, welding positions, and spotting weld defects. Students will experience hands-on lab work.
Course Outcomes Upon completion of this course, the student will be able to:
- make multiple pass welds in all positions;
- present information and ideas about specific types of weld joints as well as their structural application effectively in various contexts and formats, both written and oral;
- prepare, test, and evaluate guided bend test specimens;
- make code quality welds on plate;
- properly use and apply mathematical welding formulas; and
- read and interpret welding blueprints.
F/S (N)
|
|
-
CO 150 - Principles of Contracting Credit Hours: 3
A basic course in the organization, management and operation of a construction business. Topics include key players and relationships, contract management, estimating, contract documents, scheduling, safety, business types, risk management, building codes, procurement and inspection.
Course Outcomes At the end of the semester, the student should be able to:
- explain the organization and operations of the construction industry from the management/ownership viewpoint;
- be familiar with techniques used for planning, scheduling, estimating, bidding, controlling and analyzing construction projects; and
- be able to implement procedures, process reports and manage the legal documentation required for construction contracting.
F (N, S)
|
|
-
CO 157 - Introduction to Gas Metal Arc Welding, Metal Inert Gas Welding Credit Hours: 3
This course introduces the student to the fundamental skills of Gas Metal Arc Welding GMAW. Major topics include proper use of personal protective equipment, equipment set up, principles of operation, proper machine maintenance, component identification, process variables, and manual and automatic exercises. Laboratory time will emphasize technique and skill development.
Course Outcomes Upon completion of this course, the student will be able to:
- apply welding procedures to fabricate weldments;
- apply machining procedures to modify weldments to final drawing specifications;
- analyze blueprints and disseminate the information to build a weldment;
- examine weldment design shapes and mathematically calculate the solutions to build a weldment;
- associate the basic principles of chemistry as they apply to the welding field;
- communicate project information through written methods; and
- recognize hazards in the workplace and operate in a safe manner.
S (N)
|
|
-
CO 165 - Construction II Credit Hours: 3
A course covering the fundamental principles of materials, installation methods, safety and management used in commercial/ industrial construction. Laboratory project involves the physical application of topics discussed in lecture and computer based record keeping of labor performances in commercial/industrial construction. Course focuses on traditional construction methods used in producing concrete systems, masonry block systems, brick systems, or metal framing techniques.
Course Outcomes Upon successful completion of this course, the student will be able to:
- identify individual building components relating to foundation systems, framing systems, exterior and interior finish systems and roofing systems for commercial and/or industrial construction;
- basic knowledge with the use and layout of building materials in commercial and/or industrial construction;
- basic knowledge with the use of building codes in commercial and/or industrial construction;
- understanding how a set of commercial and/or industrial construction drawing documents are used in the industry;
- an appropriate use of construction techniques and skills to maximize the use of materials and modern tools within the construction industry;
- an ability to apply creativity in the design of systems, components or processes appropriate to lab project objectives;
- an ability to function effectively on teams;
- an ability to identify, analyze, and solve technical problems with methods of project component installations;
- an ability to communicate effectively through project assignments and notebook submissions;
- an ability to use computers with Microsoft office products;
- use of the internet to locate materials and equipment for construction; and
- use of local network printers.
F/S (N)
|
|
-
CO 185 - Principles of Mechanics Credit Hours: 3
A basic course in statics and strength of materials. The first half of this course includes basic principles of statics, force systems, centroids, center of gravity and moments of inertia of areas. An introduction to the basic concepts of structural steel and structural wood design.
Course Outcomes Upon successful completion of this course, the student will be able to:
- investigate and determine simple planer vector forces graphically and algebraically;
- investigate and determine reactions of simply supported beams;
- identify, analyze and solve technical problems;
- understand basic principles of statics, force systems, and moments of inertia; and
- investigate and determine simple wood spanning elements from tables and formulas.
Prerequisites: MT 121 F/S (N)
|
|
-
CO 210 - Drafting for Construction Credit Hours: 3
A course in basic drafting. Topics include techniques of line drawing, presentation of construction plans, details and sections by orthographics and isometrics of residential and commercial structures.
Course Outcomes Upon successful completion of this course, the student will be able to:
- exhibit basic techniques using drafting tools;
- design and introduce the underpinnings and concepts of architectural drafting;
- recognize the conventional language of drafting; and
- exhibit competence in conveying line quality and lettering techniques.
F/S (N)
This course is not required if CO212, Construction Management Internship, is successfully completed. |
|
-
CO 212 - Construction Management Internship Credit Hours: 3
The internship is designed to provide realistic hands-on training in the construction management engineering technology field with emphasis on commercial project scheduling, sub-contractor coordination, computer integrated recordkeeping, estimating, and/or construction submittal processes. This work experience, along with the academic management engineering program, will enable the student to prepare for entrance into a competitive work environment. Additionally, the internship experience will create potential employment opportunities and a bond between the student, the college, and the construction community.
Course Outcomes Upon successful completion of this course, the student will be able to:
- an appropriate mastery of the knowledge, techniques, skills, and modern tools of their discipline;
- an ability to conduct, analyze, and interpret experiments and apply experimental results to improve processes;
- an ability to function effectively on teams;
- an ability to identify, analyze, and solve technical problems;
- an ability to communicate effectively; and
- a recognition for the need for and an ability to engage in lifelong learning.
Prerequisites: 2.5 GPA minimum, completion of 12 credit hours in the Construction Management Engineering Technology department and faculty recommendation. SS (N)
This course may be taken in place of CO210, Drafting for Construction. |
|
-
CO 220 - Construction III Credit Hours: 3
A course covering the fundamental principles of design and installation of heating, cool air conditioning, ventilation, plumbing systems, electrical power and lighting systems used in the construction industry. Laboratory project encompasses the physical application of the design and installation of heating, cool air conditioning, ventilation, plumbing systems, electrical power, and lighting systems.
Course Outcomes Upon successful completion of this course, the student will be able to:
- identify individual building components relating to water and wastewater systems, mechanical systems and electrical systems for residential, commercial and/or industrial construction;
- basic knowledge with the use and layout of building materials in residential, commercial and/or industrial construction;
- basic knowledge with the use of building codes in residential, commercial and/or industrial construction;
- understanding how a set of residential, commercial and/or industrial construction drawing documents are used in the industry;
- an appropriate use of construction techniques and skills to maximize the use of materials and modern tools within the construction industry;
- an ability to apply creativity in the design of systems, components or processes appropriate to lab project objectives;
- an ability to function effectively on teams;
- an ability to identify, analyze, and solve technical problems with methods of project component installations;
- an ability to communicate effectively through project assignments and notebook submissions;
- an ability to use computers with Microsoft office products;
- use of the internet to locate materials and equipment for construction; and
- Use of local network printers.
F/S (N)
|
|
-
CO 230 - Estimating for Construction Credit Hours: 3
This is an introductory course studying various types of estimates with approximate and exact methods of quantity takeoff, methods at arriving at lump sum and unit prices, methods of itemizing preliminary and final estimates. These methods are applied to selected problems taken from various residential and commercial plans. Spreadsheets are used for recapitulation.
Course Outcomes Upon completion of this course, the student will be able to:
- develop a systematic approach to the take-off process;
- measure the work of the project in the form of a series of quantified work items;
- demonstrate the use of special computer programs for estimating and creating spreadsheets;
- use the means cost index effectively to attain prices and other information relative to the estimating process;
- quantify the general requirements of the project in such a way that the contractor can assign a monetary value to each; and
- create a portfolio (notebook) of the project.
Prerequisites: CI 100, CO 120, MT 121 F/S (N)
|
|
|
|
-
CO 250 - Principles of Structural Design Credit Hours: 3
Consists of stress and deformation, structural joints, shear and moment in beams, stresses in beams, design of beams, deflection of beams and design of columns.
Course Outcomes Upon successful completion of this course, the student will be able to:
- understand basic principles of stress and deformation in simple structural elements;
- investigate and determine shear and moments of simply supported beams;
- indentify, analyze and solve technical problems;
- investigate and determine beam deflection and bending in simple steel members; and
- understand basic principles of structural design loads and methods.
Prerequisites: CO 185 F/S (N)
|
|
-
CO 265 - Construction Management Credit Hours: 3
Planning, scheduling, controlling and analyzing progress of project or a particular operation. Items included are long-range and short-range scheduling, materials management, proper policing of an accepted schedule, treating shortages, recognizing real problems, job organization and the importance and value of meeting schedules and cost estimates.
Course Outcomes Upon successful completion of this course, the student will be able to:
- plan, schedule, control and analyze the progress of a project;
- prepare/Organize long and short range scheduling and materials management;
- manage proper policing of accepted schedule, treating shortages and recognizing real problems;
- realize job organization, importance and value of meeting the schedule and cost control;
- communicate effectively both written and orally in reports and presentations; and
- understand professional, ethical and social responsibilities.
Prerequisites: CI 100 completed; CO 150 or concurrent F/S (N)
|
|
-
CO 275 - Estimating Earthwork projects Credit Hours: 3
This course introduces engineering fundamentals for planning, selection and utilization of construction equipment as needed for earthwork projects. Methods used to excavate, compute volumes, haul and place the material in its final disposition are examined. Fundamental concepts of machine utilization are presented using standard formats for analyzing basic operational characteristics and the costs associated with equipment production.
Course Outcomes At the completion of this course, the student will be able to:
- demonstrate an understanding of construction drawings;
- demonstrate the procedure for estimating quantities for various types of excavations and earthwork projects;
- calculate project time;
- make equipment selection based on machine productivity; and
- determine probable hourly equipment costs based on depreciation, investment and maintenance costs.
Prerequisites: CI 100, CO 120, MT 121 F/S (N)
|
|
-
CO 285 - Contracts and Specifications Credit Hours: 3
A basic course in construction law and contracts with equal emphasis on interpreting and writing of construction specifications.
Course Outcomes Upon successful completion of this course, the student will be able to:
- an ability to communicate effectively through project and homework assignments;
- an ability to differentiate between types of contracts used in the construction industry;
- an understanding of the technical terms used in contracts, specifications and the construction industry;
- an ability to differentiate between multiple sections of technical specifications used in the construction industry;
- understanding of C.S.I. formats with master specifications;
- understanding of boiler plate requirements within project manuals; and
- understanding the different types of contract documents used in construction.
Prerequisites: CO 150 F/S (N)
|
|
-
CO 295 - Engineering Problem Applications Credit Hours: 4
This course applies trigonometric functions to practical engineering problems where analysis based system performance is predicted. It also introduces the student to the solution of real world statistical problems utilizing probability and its application to make decisions applicable to engineering. System performance based on analysis is predicted and methods of measurement are defined.
Course Outcomes At the completion of this course, the student will be able to:
- define methods of measurement;
- apply ratios and proportions for problem solving;
- use trigonometric processes to conduct structural analysis of a system;
- use statistical data to make analysis and prediction; and
- apply probabilities to engineering project uncertainties.
Prerequisites: CI 100, CO 185, MT 122 F/S (N)
|
Crime Scene Technology |
|
-
IN 100 - Introduction to Crime Scene Technology Credit Hours: 3
This course will concentrate on the investigation techniques and processes in the current criminal justice system. It is designed to provide students with a comprehensive understanding of criminalistics relative to criminal as well as non-criminal investigations. In depth crime scene scenarios will be used to develop student decision-making skills. Finally, the course will provide students with the fundamentals necessary to pursue advance courses in criminal justice.
Course Outcomes Upon completion of the course, the student will:
- demonstrate an understanding of the role of the criminal investigator at a crime scene;
- demonstrate an understanding of history of crime scene investigation, the preservation of evidence, the function of collection, and chain of custody, the function of the forensic laboratory; and
- be able to enter field of crime scene investigator, with a command of his/her responsibility.
F/S (C, N)
|
|
-
IN 110 - Crime Scene Photography Credit Hours: 3
Forensic photography is an essential aspect of crime scene investigation. Photographs are used in many ways to help solve crimes. The student must know proper crime photographic techniques in order to document the circumstances surrounding the crime scene. This is a technical course designed to provide a comprehensive program, while illustrating all of the important facets of photography. Since witness statements, evidence identification and crime scene reconstruction may hinge upon proper photographic images, it is imperative to be knowledgeable of every aspect of crime scene photography. This course will guide the student through the evolution of photography from its roots to the sophisticated computer imaging techniques used in law enforcement today.
Course Outcomes Upon completion of the course, the student will:
- demonstrate an insight into photography;
- demonstrate an understanding of history of crime scene photography, black/white, color and digital imaging, the function of the photography, enlargement, and court presentation; and
- be able to enter field of crime scene investigator, with a command of his/her responsibility.
F (C, N)
|
|
-
IN 200 - Fingerprint Technology Credit Hours: 3
This course is designed to familiarize students with the visualization, detection, development and recording of fingerprint impressions. Concentration on the use of fingerprints for personal identification, as well as their use in criminal investigations will be taught. This course will provide students with the opportunity to gain hands-on experience with a variety of accepted methods and techniques for processing latent fingerprints, including dusting, chemical development and the use of alternative light sources (including a black light and security laminated verifies). Additionally, the course will supply students with the necessary fundamentals to pursue advanced courses in forensic science.
Course Outcomes Upon completion of the course, the student will:
- demonstrate an understanding of the role of the criminal investigator at a crime scene;
- demonstrate an understanding of history of fingerprints, identification of fingerprint patterns, and classification of fingerprints;
- demonstrate the location of fingerprints, processing, photographing and preservation of prints;
- demonstrate the ability of make a comparison of fingerprints left at a crime scene to those of a suspect
S (C, N)
|
|
-
IN 210 - Courtroom Presentation of Evidence Credit Hours: 3
This class will provide the student with the essentials of testifying, and presentation of evidence in the courtroom. The student will study the essentials of proper presentation of evidence, charts, crime scene drawing, photographs, and fingerprints. The student will study the use of Crime and Crash Zone, ComParaprint +II and Photo Suite 8.0 photograph enlargement programs. They will also learn the task of qualifying as a court witness and participate in moot courts.
Course Outcomes Upon completion of the course, the student will:
- demonstrate an understanding of the role of the criminal investigator at a crime scene;
- demonstrate an understanding of the history of crime scene investigation, the preservation of evidence, the function of collection of evidence, chain of custody, and the function of the forensic laboratory;
- demonstrate the ability to qualifying and testify in court
S (C, N)
|
Criminal Justice |
|
-
CR 150 - Introduction to Criminal Justice Credit Hours: 3
This course is designed to provide the student with an overview of the American criminal justice system, to provide sufficient background knowledge for students to become conversant in the important concepts relating to criminal justice, and to prepare students for success in other criminal justice classes as well as for careers in public service.
Course Outcomes Upon completion of this course, the student will be able to:
- list the three phenomena of the 1960s and 1970s that stirred interest and led to changes in the American criminal justice system;
- understand the significance of the 4th, 5th, 6th, 8th, and 14th Amendments to the U. S. Constitution in the administration of justice;
- demonstrate an understanding of the key issues surrounding the need for the government to balance the maintenance of law and order versus individual rights;
- discuss the measurement and patterning of criminal behavior in America;
- name the major agencies involved in processing a person through the criminal justice system and differentiate their roles; and
- describe his/her beliefs about justice in America, as well as the triumphs and failures of the criminal justice system.
F/S (C, N, S)
|
|
-
CR 160 - Criminology Credit Hours: 3
A study of crime and society in the United States; the sociological theory of criminal behavior, indexes of crime, relationships of criminal behavior to the home institutions, race and nativity, psychopathy, culture and behavior systems.
Course Outcomes Upon completion of this course, the student will be able to:
- discuss and analyze major theories of crime causation;
- discuss the nature, extent and patterns of crime;
- discuss the criminal justice system; and
- discuss and analyze criminal behavior.
F/S (C, N, S)
|
|
-
CR 170 - Criminal Law Credit Hours: 3
A survey of the New York State and other states criminal law including definitions, terminology, application, culpability, justification, classification of crimes and sentences.
Course Outcomes Upon completion of this course, the student will be able to:
- understand criminal law in general and the New York State Penal Law in particular;
- distinguish between violations, misdemeanors and felonies;
- understand the use of force; and
- understand the terminology used in the criminal justice system.
F/S (C, N, S)
|
|
-
CR 200 - Industrial and Retail Security Credit Hours: 3
A study of crime, security and safety problems in business and industrial establishments; the control of these problems by security forces and the interrelationship of these forces and law enforcement agencies, with the goal of obtaining a New York State Security License.
Course Outcomes Upon completion of the course, the student will be able to:
- demonstrate an understanding of the role of the security officer and supervisor’s role;
- demonstrate an understanding of history of security, security measures used by industrial and retail establishments and how it relates to residential security; and
- be able to enter security work with a command of his/her responsibility.
S (C, N, S)
|
|
-
CR 210 - Deviant Behavior Credit Hours: 3
Examination of various areas of physical and mental health which bear upon public safety and well being, including the problems of alcoholism, addiction to drugs, mental illness, suicide and sexual aberrations. The objective is to provide scientifically valid guidelines so that the confrontation of those in law enforcement with the mentally ill and with social deviates will result in general benefit to the community.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate an understanding of the various theories of deviance and their application to both criminal and non-criminal behaviors;
- understand the nature and patterns of interpersonal violence;
- recognize people with mental disorders: psychotics, psychopaths, and personality disorders;
- comprehend the phenomenon of substance abuse, to include the uses, effects and addiction patterns of narcotics;
- evaluate suicide risk potential through an understanding of the variety of suicidal experiences as well as situational factors that effect suicide; and
- develop an awareness of and sensitivity to a variety of diverse lifestyles.
F/S (C, N, S)
|
|
-
CR 220 - Criminal Justice Ethics Credit Hours: 3
Course involves the study of the trends that impact the probability of human conduct. Relationships to the Declaration of Independence, U.S. Constitution, American System of Criminal Justice, Cannons of Police Ethics and Law Enforcement Code of Ethics and, resolving practical problems of an ethical nature will be studied in depth. The course is directed toward personal integrity through self-perception in a professional setting.
Course Outcomes Upon completion of this course, the student will be able to familiarize others with morality, ethics and human behavior through:
- discussion that includes developing moral and ethical behavior, predicting behavior, teaching ethics in criminal justice, criminology, and justice from criminal justice systems perspective;
- evaluating the role of the police in society, authority and power, discretion and duty, graft and gratuities and, discretion in Investigation and Interrogation; and
- analysis of ethical practices pertaining to detainment, corrections, courts, and legal defense.
F/S (C, N, S)
|
|
-
CR 230 - Criminal Investigations I Credit Hours: 3
General objectives and qualities of a criminal investigator, history of criminal investigations, and investigative steps including preliminary, follow-up and closing, rules of evidence, interviewing techniques and body language.
Course Outcomes Upon completion of this course, the student will be able to:
- understand the history of criminal investigations;
- how to conduct crime scene searches;
- handling and marking of all physical evidence recovered; and
- development of investigative techniques.
Concurrent Registration: CR 231 F (N)
|
|
-
CR 231 - Lab for CR 230 Credit Hours: 2
Implementation of forensic science techniques in criminal investigation, the handling and recording of physical evidence, crime scene photography and sketching, plastic casting, fingerprinting, (including lifting techniques and classification) surveillance technique.
Course Outcomes Upon completion of the course, the student will:
- demonstrate an understanding of the role of the criminal investigator;
- demonstrate an understanding of methods of crime scene investigation, collection of evidence, crime scene sketching, processing the crime scene for fingerprints, classification and identification of fingerprints left at scene; and
- be able to enter police work with a command of his/her responsibility.
Concurrent Registration: CR 230 F (N)
|
|
-
CR 250 - Introduction to Corrections Credit Hours: 3
This course is designed to study the history of corrections in the world. The history of correction from 2000 BC to the present will be covered. This course will cover the following areas: the American system of corrections (including prisons); penitentiary; jails; probation; parole; juvenile justice; and alternate punishment concept/usages.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate an understanding of the history of corrections and its operations today;
- comprehend the present prison system and alternatives to prison;
- differentiate the relationship between corrections, parole, probation, and how each works within the criminal justice system; and
- understand the application of the juvenile justice system and punishment, and how it differs from the adult correctional system.
F/S (C, N, S)
|
|
-
CR 260 - Criminal Courts and Procedures Credit Hours: 3
An examination of the total formal criminal process from accusation through final appeal. The jurisdiction and procedures of the magistrate, superior and appellate courts, both state and federal, are covered. The Criminal Procedure Law of New York State and Federal Law are covered in detail.
Course Outcomes Upon completion of this course, the students will acquire an understanding of criminal procedure law in general and New York State Criminal Procedure Law in particular through:
- the evaluation of the law, critically, relative to fairness, application and access to justice;
- the analysis of criminal procedure doctrine;
- the explanation of the various stages, components and participants that comprise NYS Criminal Procedure Law; and
- the relationship between the NYS and US Constitutions.
F/S (C, N, S)
|
|
-
CR 270 - Police Administration Credit Hours: 3
An intensive study of the internal arrangement and functions of local police departments: Organizational and leadership problems, planning and research, inspection procedures, personnel matters, training and public relations, operational services, patrol, investigations, vice and youth.
Course Outcomes Upon completion of this course, the student will be able to:
- have the knowledge to articulate the many issues confronting contemporary police and law enforcement agencies;
- demonstrate an understanding of the history of American policing and its continuous evolution and the development of police administration;
- understand the complexities of contemporary policing;
- articulate the nuances of rural, suburban, and urban policing;
- understand the issues confronting police chiefs of small, medium, and large police agencies; and
- evaluate the various styles of leadership present in American policing.
S (N)
|
|
-
CR 290 - Criminal Investigations II Credit Hours: 3
This course is designed to clarify the use of force, arrest procedures, probable cause, searches and seizures. Also the course will cover landmark United States Supreme Court cases and mock crime scenes set up students outside.
Course Outcomes Upon completion of this course, the student will be able to:
- understand search and seizers and the major cases settled by the United States Supreme Court;
- article 35 of the NYS Penal Code covering the use of force; and
- investigate crime scenes, collect evidence, take important crime scene notes and complete a sketch of the crime scene.
S (N)
|
|
-
CR 299 - Work/Study Seminar Credit Hours: 3
Students are placed at a cooperating worksite for the purpose of gaining practical experience in the criminal justice system. Requirements for the course include a combination of volunteer hours at a worksite and classroom instruction. Class instruction will provide students a chance for discussions of worksite and professional issues. .
Course Outcomes Upon completion of this course, the student will be able to:
- have a working knowledge of the agency they have volunteer for and how it interfaces with the criminal justice system i.e.: law enforcement, courts, and corrections;
- relate relevant course content for CR150, CR160, CR170 and CR260 to the agency they volunteered;
- have a better understanding and experience the actual practice of course content to law enforcement, courts and corrections;
- establish a resource list for mentor, employment recommendations and work experience; and
- have a working knowledge of investing employment income to generate lawful income to avoid the pitfalls of corruption, bribes, etc. that sometimes compromise criminal justice employees.
F/S (C, N, S)
Students must have a minimum of a 2.0 in all prerequisite courses. |
Culinary Arts |
|
-
HC 102 - Introduction to Culinary Arts Credit Hours: 4
An interactive overview of contemporary culinary arts and working in a professional kitchen. Selected readings, movies, practical demonstrations by and discussions with area professionals will introduce the student to the world of the professional chef.
Course Outcomes Upon completion of this course, the student will be able to:
- identify and discuss both the positive and negative aspects of a career in food preparation and service in the hospitality industry;
- recognize the different career paths and opportunities open to students pursuing a culinary career;
- discuss restaurant criticism and the media’s perception of the professional chef in print and film;
- identify the characteristics of good work ethic in the hospitality industry;
- observe the preparation and sample dishes by department instructors and area chefs; and
- use Internet resources for professional development.
S (C, N)
|
|
-
HC 110 - Hospitality Mathematics Credit Hours: 3
A course designed to develop the math skills necessary to complete measurement conversion, portion control and costing, recipe conversion and production control forms used in the industry. Standard systems, including computerized ones used to perform these functions will be introduced.
Course Outcomes Upon completion of this course, the student will be able to:
- accurately calculate ingredient cost based on type of food, container, trim loss and weight to volume or volume to weight conversion;
- accurately calculate recipe portion costs using accurate ingredient cost information; and
- use at least two different methods for calculating profitable menu prices.
Using the computer:
- produce “hard copy” of recipes featuring detailed preparation instructions or cost analysis information;
- add new ingredient information to program inventory, including proper price, purchase unit information and recipe unit information; and
- generate a “shopping list” of ingredients for selected recipes.
Prerequisites: Math placement test score of college algebra. Corequisites: MT 003 or MT 006 F/S (C, N)
|
|
-
HC 114 - Culinary Arts Basic Skills Credit Hours: 4
The basic skills required for the preparation, presentation and storage of hot foods will be presented. Emphasis is placed on an understanding of the various cooking methods used in the commercial kitchen. The student will begin to acquire a sense of comfort and control in the kitchen, as well as the discipline to maintain a commercial food preparation environment in a safe and sanitary manner.
Course Outcomes Upon completion of this course, the student will be able to:
- maintain a safe and sanitary work environment, based on professional standards;
- identify the ingredients and preparation procedures for brown and white stocks;
- describe mise en place;
- list the moist- and dry-heat cooking methods;
- identify the ingredients and preparation procedures for clear, thickened and cream soups;
- identify composition, structure and basic quality factors for meats, poultry, seafood and vegetables; and
- designate and demonstrate appropriate cooking methods for particular meats, poultry, seafood and vegetables based on their composition, structure and quality factors.
Corequisites: HR 136 Concurrent Registration: HC 116 F (C, N)
|
|
-
HC 116 - Introduction to the Pantry and Garde Manger Credit Hours: 4
Acquaints the student with preparation and storage techniques necessary for the operation of a commercial kitchen’s pantry area. Sandwich and hors d’oeuvre production is practiced, as is the attractive presentation of various salads. Introductory level food carving and display techniques are demonstrated, with individual practice encouraged. Other topics will include salad dressings, breakfast preparations and dairy products, with a sensory evaluation of an international assortment of cheeses.
Course Outcomes Upon completion of this course, the student will be able to:
- prepare appetizers, hors d’oeuvres and sandwiches to the specifications of the instructor and the text;
- demonstrate the proper method for handling and storage of leafy greens, fruits and vegetables, breads and dairy products;
- prepare various Charcuterie items including sausages and terrines to the specifications of the instructor and the text;
- identify the major classifications of fresh and cultured dairy products, including types of cheeses produced by various ripening methods and strains of mold;
- identify and demonstrate various methods for the production of ice creams, sorbets, granites and their accompaniments;
- properly brew coffee and tea for table service;
- plan and attractively display a selection of hors d’oeuvres and charcuterie to be judged on menu balance, craftsmanship, portion size and degree of difficulty;
- demonstrate the successful preparation of an emulsion such as mayonnaise, and other types of salad dressings; and
- show an increased level of comfort in the kitchen environment, including better knife skills, proper sanitary and safety techniques, and awareness of maintaining a professional work environment.
Corequisites: HR 136 Concurrent Registration: HC 114 F (C, N)
|
|
-
HC 118 - Culinary Nutrition Credit Hours: 2
An introductory course with emphasis on the practical and systematic approach to delivering the nutritional essentials to culinary professionals. There is special emphasis on interactions among menu planning selection, the handling and preparation techniques of food in meeting current nutritional guidelines. The topic will discuss the basic nutrients in combination with applications in food preparation. This course meets the American Culinary Federation Nutritional requirement for certification.
Course Outcomes Upon completion of this course, the student will be able to:
- identify the basic nutrients, list their requirements, and select appropriate menu items in adequate amounts to provide these;
- prepare and evaluate menus and food intake records in terms of current nutrition knowledge; and
- identify customer clientele, needs, and preferences for various food selections based upon established nutritional criteria.
F (C, N)
|
|
-
HC 122 - Menu Design Credit Hours: 3
This course is designed to acquaint the student with the art of menu planning. Topics will include menu design as it relates to the control of food and labor cost, the use of equipment and the merchandising of food and beverage.
Course Outcomes Upon completion of this course, the student will be able to:
- understand the beginnings and growth of the foodservice industry;
- identify various types of foodservice operations;
- differentiate between the different types of operations;
- identify economic and social trends likely to impact the future of the foodservice industry;
- identify and characterize the various menus offered in the foodservice industry;
- idetify the organization structure of a menu;
- identify tools needed to plan a menu;
- explain how cost factors affect menu planning;
- describe cost factors unique to commercial and nonprofit operations;
- characterize the most common pricing techniques used in the foodservice industry; and
- identify the basic requirements to develop a menu.
S (C, N)
|
|
-
HC 124 - Introductory Hot Foods Credit Hours: 4
A selection of soups, vegetables, starches and entree items are prepared to familiarize the students with menu items served in the hospitality industry. Lecture demonstration and student participation will focus on the production and attractive presentation of hot food items. Theory and practice in cooking methods such as deep frying, poaching, sautéing, broiling and roasting will be covered. The student will be introduced to the requisitioning and costing done by a sous or executive chef.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the proper use of tools and operation of equipment needed for food preparation, production and storage;
- demonstrate safe and sanitary procedures for food preparation and storage;
- fulfill the requirements of the laboratory maintenance schedule;
- demonstrate an increased proficiency in the culinary techniques (organization, knife, mise en place) used in hot food preparation over introductory level skills;
- employ elementary menu planning techniques, select a starch and vegetable accompaniment for a given entrée;
- calculate the proper amounts for the entire class of all ingredients needed for the recipe, and requisition them from the storeroom;
- write a clear, accurate recipe for the starch and vegetable items then complete a recipe cost analysis report for these recipes;
- identify proper preparation techniques, storage and handling methods and characteristics for fruits, vegetables, tubers and roots, and farinaceous ingredients; and
- successfully demonstrating to chef instructors handling, cooking and serving seasonal vegetables, starch and\ or potato, and a basic entrée in the time set.
Prerequisites: HC 114, HC 116 S (C, N)
|
|
-
HC 126 - Intermediate Hot Food Credit Hours: 4
A continuation of the study and practice of preparation and presentation of hot food items being served in commercial establishments. A research-based awareness of current trends in ingredients and preparation techniques will lead to laboratory practice including, but not limited to, spa cuisine, pasta dishes and the use of exotic fruits and vegetables. The student’s work will culminate in a practical food preparation exam.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the proper use of tools and operation of equipment needed for food preparation, production and storage;
- demonstrate safe and sanitary procedures for food preparation and storage;
- fulfill the requirements of the laboratory maintenance schedule;
- demonstrate an increased proficiency in the culinary techniques (organization, knife, mise en place) used in hot food preparation over introductory level skills;
- employ elementary menu planning techniques, select a starch and vegetable accompaniment for a given entrée;
- calculate the proper amounts for the entire class of all ingredients needed for the recipe, and requisition them from the storeroom;
- write a clear, accurate recipe for the starch and vegetable items then complete a recipe cost analysis report for these recipes;
- identify proper preparation techniques, storage and handling methods and characteristics for fruits, vegetables, tubers and roots, and farinaceous ingredients; and
- successfully demonstrating to chef instructors handling, cooking and serving seasonal vegetables, starch and/or potato, and a basic entrée in the time set.
Prerequisites: HC 124 S (C, N)
|
|
-
HC 128 - Introduction to Baking Credit Hours: 4
An introduction to the bakery, its equipment and methods used in production. Topics to be covered will include quick breads, yeast breads, pies, cakes, tortes, along with holiday specialty products commonly prepared in the bake shop.
Course Outcomes Upon completion of this course, the student will be able to:
- recognize, operate and care for the equipment used in the bake shop;
- recognize all ingredients to produce baked products for operation outlets;
- maintain professional standards in production, as well as maintaining a safe and sanitary work space;
- understand formulas and how to correct and adjust their size; and
- learn the fundamentals of baking.
F/S (C, N)
Non majors allowed; permission of the instructor is required. |
|
-
HC 129 - Intermediate Baking Credit Hours: 4
A continuation of the baking methods and formulas introduced in Introduction to Baking. Topics to be covered included more advanced yeast bread, pies, tarts, cakes, cheesecakes and custards along with bakery products commonly served at breakfast.
Course Outcomes Upon completion of this course, the student will be able to:
- recognize, operate and care for the equipment used in the bake shop;
- recognize all ingredients to produce baked products for operation outlets;
- maintain professional standards in production, as well as maintaining a safe and sanitary work space;
- understand formulas and how to correct and adjust their size; and
- demonstrate an observable improvement in workmanship compared to items produced in HC 208.
Prerequisites: HC 128 Concurrent Registration: HC 130 F/S (C)
|
|
-
HC 130 - Advanced Pastries and Cakes Credit Hours: 4
A continuance of the baking methods and formulas introduced in introductory and intermediate baking classes. Topics to be covered include advanced work in pastries, cakes, icings, and decorations. Production techniques for a variety of baked and frozen preparations will be presented. An introduction to chocolate and sugar work will be presented, as well as elementary concepts of plated dessert presentation.
Course Outcomes Upon completion of this course, the student will be able to:
- recognize, operate and care for the equipment used in the bake shop;
- recognize all ingredients to produce baked products for operation outlets;
- maintain professional standards in production, as well as maintaining a safe and sanitary work space;
- understand baking formulas and how to correct and adjust their size; and
- demonstrate an observable improvement in workmanship compared to items produced in HC 210.
Concurrent Registration: HC 129 F/S (C)
|
|
-
HC 132 - Chocolate Confections Credit Hours: 3
A course designed to introduce students to the comprehensive scope of chocolate preparation. Emphasis will be on tempering, molding, sculpting and preparing chocolate desserts. The student will learn the fundamentals of the science of chocolate, moving on to tempering, where they practice hand tempering high grade chocolate coverture. Students learn to produce a wide range of chocolate candies including ganache fillings, hand dipped candies, molded bonbons and truffles.
Course Outcomes Upon completion of this course, the student will be able to:
- understand the fundamentals of the science of chocolate;
- temper chocolate;
- make a wide range of chocolate candies including ganache fillings, hand-dipped candies, molded bon-bons, and truffles;
- apply updated methods of making traditional recipes and efficient production methods utilizing proper equipment; and
- properly clean and store chocolate candy molds and other equipment used in chocolate candy making.
F/S (C)
|
|
-
HC 138 - Specialty Cakes Credit Hours: 3
In this course, the students learn a wide variety of cake decorating techniques including detailed piping techniques, French buttercream frosting, rolled fondant cake covering and decorations specifically tailored for cakes.
Course Outcomes Upon completion of this course the student will be able to:
- evaluate rolled fondant techniques to cover cakes;
- identify various fillings such as butter cream, ganache, meringue, mousse, flavored whipped cream, pastry cream, and different glazes;
- understand how to apply the aforementioned fillings through various methods of constructing cakes using efficient production methods;
- evaluate methodologies of combining flavor, texture and taste. This comprehension will allow students to develop the ability to create their own flavor and texture combinations, as well as recipes;
- demonstrate piping techniques using both buttercream and royal icing from basic to advanced piping skills; and
- create professional celebration cakes.
S (C)
|
|
-
HC 143 - Working in the Learning Garden II Credit Hours: 3
This is a continuation course of HC 142, Working in the Learning Garden I. In this course, the student will continue to learn the fundamentals of gardening. Emphasis will be on garden to plate sustainability and food preservation. The learning garden will provide the skills needed to successfully plant, grow and maintain a productive garden.
Course Outcomes Upon completion of this course, the student will be able to:
- analyze square foot gardening, comparing the number of plants needed for the space provided;
- distinguish between pre-started plants and seeds. Identify what plants are most effective to use;
- demonstrate the understanding of composting and the critical role worms play in a successful garden;
- demonstrate the knowledge of soil provisions, water, toners and plant foods necessary to continuously improve soil conditions;
- develop an understanding of pollination and its necessary role to create the fruit and kill harmful bugs; and
- develop menus based on seasonal crops grown.
SS (C)
|
|
-
HC 230 - Food and Labor Cost Control Credit Hours: 3
An overview of concepts, terminology and mathematical procedures involved in cost analysis and budgeting. Systems for control of direct and indirect costs of operation will be examined. Students will operate their own restaurant, making cost decisions through the use of computer simulation concerning the menu, labor costs and other direct costs of operation.
Course Outcomes Upon completion of this course, the student will be able to:
- identify the types of costs incurred in hospitality operations;
- understand the purpose of budgeting and the steps to take in the development of a budget;
- calculate food cost percentage and evaluate food cost results;
- identify cost, volume & profit relationships; and
- describe the techniques used to control labor cost as well as other types of costs.
Prerequisites: HC 110 F (C, N)
|
|
-
HC 232 - Wines, Beers and Spirits Credit Hours: 3
A study of the major alcoholic beverages related to the culinary arts. A major portion of the instruction will be devoted to the study and sensory evaluation of wines from Europe, America, and Southern Hemisphere. Proper storage and service will be discussed, as will production factors and procedures. Techniques using alcoholic beverages in food preparation will be demonstrated and practiced. A materials fee will be collected by the instructor.
Course Outcomes Upon completion of this course, the student will be able to:
- identify the main categories and characteristics of alcoholic beverages, with an emphasis on wine;
- explain the factors necessary for the quality production of various types of alcoholic beverages, particularly foreign and Domestic wines;
- properly serve wines, beers and spirits;
- experience various cooking methods using wines, beers and spirits;
- visit and compare two or more regional wineries;
- understand the laws and moral obligations concerning the service of alcoholic beverages; and
- prepare a wine list for a predetermined type of restaurant.
F (C, N)
|
|
-
HC 234 - Advanced Food Preparation and Service I Credit Hours: 4
Advanced courses, building upon the basics established in previous course work. In these courses, the student will refine their techniques used in food preparation and service in an actual business setting. Students will rotate through various workstations in the E.M. Statler Dining Room, Statler Erie Room, and campus cafeteria food service operations.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the proper operation, cleaning, and maintenance of commercial foodservice equipment;
- perform proper sanitation and safety procedures;
- recognize, setup, and execute proper food service techniques in a quantity food production facility;
- produce quality menu items for guests in a quantity food production facility;
- plan and produce a cycle menu based on the New York State Child and Adult Care Food Program (CACFP);
- recognize, setup, and execute proper food service techniques in a café/coffee shop setting;
- produce quality home replacement meals; and
- project themselves as hospitality professionals to guests and each other.
Prerequisites: HC 126, HC 128 Corequisites: HC 236 F (C, N)
|
|
-
HC 236 - Advanced Food Preparation & Service II Credit Hours: 4
Advanced courses, building upon the basics established in previous course work. In these courses, the student will refine their techniques used in food preparation and service in an actual business setting. Students will rotate through various workstations in the E.M. Statler Dining Room, Statler Erie Room, and campus cafeteria food service operations.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the proper operation, cleaning, and maintenance of commercial foodservice equipment;
- perform proper sanitation and safety procedures;
- recognize, setup, and execute proper food service techniques in a quantity food production facility;
- produce quality menu items for guests in a quantity food production facility;
- plan and produce a cycle menu based on the New York State Child and Adult Care Food Program (CACFP);
- recognize, setup, and execute proper food service techniques in a café/coffee shop setting;
- produce quality home replacement meals; and
- project themselves as hospitality professionals to guests and each other.
Corequisites: HC 234 F (C, N)
|
|
-
HC 237 - Retail Bake Shop Credit Hours: 4
This course is conducted on campus and in the retail bake shops. It is planned for the students who have completed the first semester course work in the baking program. The students will prepare a variety of products for sale and use within the college’s retail operations. Practical experience in retail bake shop operations, including production, marketing, management, costing and sales is included.
Course Outcomes Upon completion of this course, the student will be able to:
- successfully staff and operate a retail bake shop facility;
- identify and respond to or correct special problems encountered in the development and opening of a retail bake shop, such as, but not limited to, construction, health and operating codes;
- create, implement and correct, if necessary, preparation and production control systems;
- identify and demonstrate proper communication between staff, customers and suppliers; and
- successfully and efficiently conduct any preparation or transaction needed for the successful operation of the facility and the satisfaction of the customer.
Prerequisites: HC 130 Corequisites: HC 239 F/S (C)
|
|
-
HC 238 - Classical Bake Shop Credit Hours: 4
This course emphasizes the production methods used in a classical bake shop, with special direction given to large quantity production. Desserts and pastries served in exclusive fine restaurants will be prepared along with the production of classical centerpieces.
Course Outcomes Upon completion of this course, the student will be able to:
- recognize, operate and care for the equipment used in the bake shop;
- recognize all ingredients to produce baked products for operation outlets;
- maintain professional standards in production, as well as maintaining a safe and sanitary work space;
- understand formulas and how to correct and adjust their size;
- produce classical centerpieces made from cooked sugar, nouget, pastilage, chocolate, marzipan and gingerbread;
- produce tortes, specialty doughs, creams, frozen desserts; and
- present pastry buffets.
Prerequisites: HC 128 F/S (C, N)
|
|
-
HC 239 - Bake Shop/Field Experience Credit Hours: 4
This course is conducted in local retail bake shops, restaurants and commissaries in the Western New York area. It is planned for students who have completed the first semester course work in their program. The course instructors assign the student an operation. They meet with a representative of their assigned operation to discuss their career goals and set up a field experience schedule.
Course Outcomes Upon completion of this course, the student will be able to:
- experience various assigned baking and service duties in a commercial bake shop or other operation;
- experience and practice preparation and production control systems;
- develop and improve communication skills; build positive relationships with staff;
- collaboratively solve problems specific to the operation; and
- apply the fundamentals of baking in a commercial setting.
Prerequisites: HC 130 Corequisites: HC 237 F/S (C)
|
|
-
HC 240 - Kitchen Design and Layout Credit Hours: 3
A course designed to show the relationship of activities as they affect the construction and operation of a food service facility. Emphasis will be placed upon the development of an efficient kitchen, work center, dining and support system. Work simplification in and around the work center will be presented along with the selection, purchase and placement of equipment. A major component will be the design of a food service operation by the student.
Course Outcomes Upon completion of this course, the student will be able to:
- understand the uses and characteristics of various food service facilities;
- understand the planning process and the interaction of the members of the planning team;
- understand the importance of the prospectus and its use;
- prepare a feasibility study for planning a food service facility;
- understand the relationship between the functions performed in the kitchen, service areas, and other areas in the typical food service facility;
- design a workplace for maximum efficiency and productivity;
- determine the specific equipment requirements for food service facilities;
- understand and determine space requirements needed;
- understand the different types of common food facilities layouts;
- understand the support and physical conditions as they affect the facility and its workers; and
- understand the principles of motion economy and work simplification in the work place.
S (C, N)
|
|
-
HC 242 - American Regional Cuisine Credit Hours: 4
The course is designed to acquaint the student with the international and classical cuisines of the world. The history of the cuisine, as well as the preparation and presentation of native foods will be stressed. This course will take place in the E.M. Statler Dining Room.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the proper use of tools and operation of equipment needed for food preparation, produc¬tion and storage;
- demonstrate safe and sanitary procedures for food preparation and storage;
- fulfill the requirements of the laboratory mainte¬nance schedule;
- conduct searches of the World Wide Web to obtain information about various cuisines of the world;
- identify the primary cooking techniques, ingredients and seasonings of major cuisines;
- properly complete all paperwork including requisitions, inventory and production sheets and cost analysis. Also, accurately report cash receipts and reconciliation of the cash drawer;
- demonstrate an increased proficiency in the culinary techniques used in hot and cold food preparation over introductory level skills;
- courteously and professionally serve all dining room guests, using the standards of the National Restaurant Association; and
- courteously and professionally manage and maintain the Dining Room including: pleasantly greeting guests, seating, accurately filling out order dupes and guest checks, operating the cash register and making change.
Prerequisites: HC 126, HC 128 Corequisites: HC 244, HC 246 F/S (C, N)
|
|
-
HC 244 - Classical and International Cuisine Credit Hours: 4
The course is designed to acquaint the student with the international and classical cuisines of the world. The history of the cuisine, as well as the preparation and presentation will be stressed. Service in the style appropriate will be a major component. This course will take place in Statler Dining Room facilities.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the proper use of tools and operation of equipment needed for food preparation, produc¬tion and storage;
- demonstrate safe and sanitary procedures for food preparation and storage;
- fulfill the requirements of the laboratory mainte¬nance schedule;
- conduct searches of the World Wide Web to obtain information about various cuisines of the world;
- identify the primary cooking techniques, ingredients and seasonings of major cuisines;
- properly complete all paperwork including requisitions, inventory and production sheets and cost analysis. Also, accurately report cash receipts and reconciliation of the cash drawer;
- demonstrate an increased proficiency in the culinary techniques used in hot and cold food preparation over introductory level skills;
- courteously and professionally serve all dining room guests, using the standards of the National Restaurant Association; and
- courteously and professionally manage and maintain the Dining Room including: pleasantly greeting guests, seating, accurately filling out order dupes and guest checks, operating the cash register and making change.
Prerequisites: HC 126, HC 128 Corequisites: HC 242, HC 246 F/S (C, N)
|
|
-
HC 246 - Banquet and Buffet Management Credit Hours: 4
A study of the organization and artful presentation of buffets and catered events. Topics to be covered include menu selection, layout, preparation and presentation of meats, poultry, seafood, vegetables, salads, hors d’oeuvres, charcuterie and baked goods. Decorative pieces, including ice carvings, and garde manger work will be included in the buffet presentations.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate appropriate workplace behavior including: punctuality, initiative, planning and anticipation of the work required, acknowledgement and eager participation in achieving the goals of the operation;
- demonstrate a professional level of competence with regard to food handling, cooking, service and storage;
- properly plan the layout of a dining room for buffet service with respect to ease and efficiency of service, customer comfort, traffic flow and safety; and
- organize and set a buffet table with respect to organization, ease and efficiency of service and profitability, using attractive and appropriate display techniques.
Prerequisites: HC 126, HC 128 Corequisites: HC 242, HC 244 F/S (C, N)
|
|
-
HC 248 - Culinary Purchasing Credit Hours: 3
A study of distribution systems, factors which affect distribution systems, the general purchasing function of management, and the basic principles of selection and procurement. An identification of the selection factors used in the purchasing of food, beverages, operating supplies, services, equipment, and furnishings for use in hotels and restaurants.
Course Outcomes Upon completion of this course, the student will be able to:
- understand the channels of distribution involved in hotel/restaurant purchasing;
- recognize issues, problems, concerns, laws, and legal considerations involved in the purchasing function;
- recognize the role of purchasing within a hotel/restaurant operation and its relation to suppliers;
- recognize and utilize basic purchasing principles and buying techniques used within the hotel restaurant industry; and
- understand the factors used in the purchasing of food, beverages, supplies, equipment, furniture, and services for a hotel operation.
F/S (C, N)
|
|
-
HC 250 - Catering and Special Events Credit Hours: 3
This course is designed to teach the fundamentals of creating and planning a successful event. Emphasis will be on establishing the customer’s goal, budget planning, food and drink, entertainment and the risk and safety factors involved in creating an event.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate understanding in the use of sales techniques;
- present a wide range of event plans and their budgets; and
- acquire discernment in the establishment of the customer’s goals in having the event.
S (C,N)
|
Culinary Medicine |
|
-
CM 201 - Culinary Medicine I Credit Hours: 4
Presented in Culinary Medicine I will be foundational information in nutrition based on the Mediterranean Diet principles as they apply to basic food selection and preparation.
Meal planning, sanitation, basic kitchen skills, portion sizes, food labels, vegetarian and vegan diets, as well as several current topics of interest will be addressed. This introductory course will be presented in the classroom followed by application in the food lab.
Course Outcomes Upon completion of the course, the student will be able to:
- demonstrate the ability to properly read, follow a recipe, and prepare food utilizing basic kitchen skills and proper sanitation guidelines;
- produce and evaluate food items and recipes prepared for taste, ease of preparation, and Mediterranean diet appropriateness;
- critically evaluate recipes for adherence to the Mediterranean diet and propose appropriate adjustments to align the recipe with the Mediterranean diet;
- demonstrate how the Nutrition Facts label can be used to make healthier food choices based on macronutrient content and portion size;
- utilize and incorporate foods that are foundational to a Mediterranean diet in meal planning;
- demonstrate an understanding of reducing, removing, or replacing saturated and trans fats in food preparation; and
- through discussion and food presentation, demonstrate the ability to incorporate the application of the Mediterranean diet in circumstances of a specific health need.
|
|
|
|
-
CM 203 - Cooking with Cannabis Credit Hours: 4
The use of cannabis in foods has increased in the past decade. This course will explore the history, science, safety, and consumption of cannabis as it relates to health. Meal planning, sanitation, basic kitchen skills, portion sizes, various diets, as well as several current topics of interest will be addressed. This introductory course will be present in the classroom followed by application in the food lab.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate the ability to properly read, follow a recipe, and prepare food utilizing basic kitchen skills and proper sanitation guidelines.
- produce and evaluate food items and recipes prepared for taste and ease of preparation and cannabis/hemp appropriateness.
- critically review the key legal issues surrounding cannabis/hemp for therapeutic use.
- demonstrate how to properly cook with cannabis/hemp.
- summarize evidence-based uses for cannabis/hemp.
- identify the risks and benefits associated with cannabis/hemp.
|
Dance |
|
|
|
|
|
|
|
|
|
|
|
-
DN 111 - Beginning Tap Dance Credit Hours: 3
Designed for the first year student in tap dancing introducing the fundamental principles and technique of tap dancing combined with rhythm and jazz for modern movement and aesthetic approach to dance as an art form.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate a command of tap dance terminology;
- demonstrate a knowledge of introductory level of tap dance warm-ups;
- apply rhythms, beats, and general movements to choreographed floor progressions;
- choreograph and perform an original dance routine to music or routine taught by instructor performed to music;
- recognize his/her gain in self-awareness and confidence through mastering better control over his/her body actions/expressions; and
- recognize an appreciation of dance both as an art form and for personal enjoyment.
F/S (C)
|
|
-
DN 112 - Intermediate Tap Dance Credit Hours: 4
Designed for students who have some previous dance experience and who want to further their skills in tap dancing. The fundamental principles and technique of tap dancing, combined with rhythm and jazz, for modern movement and aesthetic approach to dance as an art form will be covered.
Course Outcomes Upon completion of this course, the student will be able to:
- demonstrate a command of tap dance terminology;
- demonstrate a knowledge of introductory level of tap dance warm-ups;
- apply rhythms, beats, and general movements to choreographed floor progressions;
- choreograph and perform an original dance routine to music or routine taught by instructor performed to music;
- recognize his/her gain in self-awareness and confidence through mastering better control over his/her body actions and/or expressions; and
- appreciate dance both as an art form and a means of personal enjoyment.
F/S (C)
|
Dental Assisting |
|
|
|
-
DS 110 - Dental Biomedical Sciences Credit Hours: 2
This course provides basic knowledge of the external and internal structures of the head and neck as related to dentistry. Bones, muscles, nerves, blood vessels and glands are emphasized, as well as landmarks of the face and oral cavity. Detailed study of the dentition includes tooth names, surfaces, morphology, functions, numbering systems and dental charting, in addition to the embryologic and histologic development and eruption of the teeth.
Course Outcomes At the completion of this course, the student should be able to:
- list and identify tooth names, surfaces, numbers, anatomical landmarks and functions;
- compare and contrast the primary and permanent dentitions and eruption schedules;
- accurately record the dentition and dental restorations in a dental chart;
- list and identify the external and internal landmarks of the face and oral cavity;
- identify and locate the salivary glands, lymph node sites, and sinuses of the head and neck and their functions;
- locate and identify the major bones, muscles, nerves, and blood vessels of the head and neck as pertaining to dentistry;
- identify the components of the temporo-mandibular joint and their functions;
- describe the stages of pregnancy and embryologic development of the teeth and oral cavity;
- describe the tooth tissues and their components; and
- name the parts and functions of the periodontium.
Corequisites: All first-semester Dental Assisting courses. F (N)
|
|
-
DS 120 - Dental Sciences Credit Hours: 2
The importance of proper diet and nutrition to overall health and its impact on the oral cavity is explored, with emphasis on the relationship of carbohydrates to the decay process. Students learn about the disease process and to differentiate and identify normal and pathologic conditions of the oral cavity. The role of pharmacology in dentistry is discussed along with the importance of the patient medical history. Includes the recognition and response to medical emergencies in the dental office.
Course Outcomes At the completion of this course, the student will be able to:
- name the components and recommendations of the Food Guide Pyramid;
- discuss the U.S. Food Guidelines;
- explain the effects of diet and nutrition on the oral cavity;
- explain the relationship of cariogenic food to the decay process;
- list the major nutrients, their functions, and sources;
- recognize signs and symptoms of eating disorders and the effect on the oral cavity;
- interpret information located on food labels;
- adapt ethnic, religious and alternative diets into the framework of a balanced diet;
- list the eight sources of information utilized to form a final diagnosis;
- differentiate between normal and abnormal conditions of the oral cavity;
- describe the signs, symptoms and steps of the inflammatory process;
- describe the classifications of oral lesions;
- identify lesions according to the placement, appearance and medical history;
- describe the types and known causes of orofacial developmental disorders;
- describe the oral implications of HIV/AIDS;
- list the warning signs and appearances of oral cancer;
- describe the guidelines of prescription writing and pharmacy calls;
- utilize various drug reference materials to access information;
- list and describe the drug schedules under Controlled Substances Act;
- name the routes of drug administration and phases of drug activity in the body;
- list the potential adverse effects of drug usage;
- demonstrate an understanding of the drugs used in dentistry;
- describe the classic signs and symptoms of a medical emergency;
- discuss the methods of response to common medical emergencies in the dental office; and
- list the basic items to include in an office emergency kit.
Corequisites: All first-semester Dental Assisting courses. F (N)
|
|
-
DS 130 - Dental Laboratory Procedures Credit Hours: 1.5
This laboratory course provides the dental assisting student with the opportunity to manipulate clinical and laboratory materials and fabricate a variety of dental products used in preventive and restorative dentistry. Emphasis is placed on knowledge of dental materials, fabrication techniques, equipment usage and laboratory safety rules.
Course Outcomes At the completion of this course, the student should be able to:
- discuss safety precautions and infection control procedures to be observed in the dental laboratory;
- list the basic types of dental laboratory equipment and their uses;
- identify the classifications and uses of waxes in dentistry;
- pour, trim and mount dental models using various gypsum products;
- prepare reversible and irreversible hydrocolloid impression materials;
- fabricate custom fit mouthguards and bleaching trays;
- fabricate and fit provisional restorations;
- construct maxillary and mandibular custom trays; and
- debride and polish fixed and removable dental prostheses and appliances.
Corequisites: All first-semester Dental Assisting courses. F (N)
|
|
-
DS 150 - Dental Assisting II Credit Hours: 4
This course provides an overview of the dental specialties and their role in general dentistry while providing the information and skills necessary to assist during related chairside procedures. Emphasis is placed on the expanded functions delegable to the New York State-licensed dental assistant.
Course Outcomes At the completion of this course, the student should be able to:
- develop an individualized caries prevention program for a dental patient;
- perform supportive services delegable to the NYS licensed dental assistant;
- demonstrate knowledge of instruments and materials as related to the recognized dental specialties; and
- assist with dental specialty procedures.
Prerequisites: All required first-semester Dental Assisting courses. Corequisites: All required second-semester Dental Assisting courses. S (N)
|
|
-
DS 160 - Dental Practice Management Credit Hours: 2
This course provides an understanding of the basic skills required for the daily business operations of a dental practice. Ethics and the law pertaining to dentistry are explored. Students are instructed in resume writing, interview techniques and the credentialing process in preparation for entry into the workforce.
Course Outcomes At the completion of this course, the student should be able to:
- understand the basic day-to-day operations of the dental business staff;
- communicate effectively with patients, staff, and business associates through oral and written communications;
- be familiarized with computer usage and business technology in the dental office;
- describe methods of external and internal practice marketing;
- maintain practice records and files;
- file dental charts utilizing the indexing rules for alphabetic filing;
- schedule patient appointments for maximum productivity;
- list the steps of an effective recall system;
- receive payments, post to patient accounts, and prepare a bank deposit;
- understand the billing and collection process for patient accounts;
- maintain an office inventory system;
- understand the steps to third party reimbursement;
- prepare a cover letter, resume, and interview strategy;
- describe professional conduct during employment;
- describe the application of ADAA Code of Ethics to daily practice;
- maintain the patient’s right to privacy according to HIPAA standards;
- recognize the legal responsibilities of the dental team to the patient;
- identify the risk factors and precautions necessary to prevent litigation against dental personnel; and
- identify, analyze and apply problem solving skills in the clinical externship experience.
Prerequisites: All required first-semester Dental Assisting courses. Corequisites: All required second-semester Dental Assisting courses. F (N)
|
|
-
DS 170 - Dental Assisting Externship Credit Hours: 3
This course emphasizes the practical application of the dental assisting skills obtained throughout the program. Students will be assigned to local dental health care facilities to participate in patient treatment under the guidance of licensed professionals. Students will acquire hands-on experience in clinical, laboratory and administrative procedures and complete a journal of externship activities. Seminars with the class and course instructor will be conducted periodically to review externship experiences.
Course Outcomes At the completion of the externship, the student should be able to:
- interact in a professional and ethical manner with patients and colleagues;
- perform the administrative skills required of a certified dental assistant;
- perform the clinical skills required of a certified dental assistant;
- perform the laboratory skills required of a certified dental assistant;
- complete requirements for national certification and NYS licensure; and
- obtain employment in an entry-level position as a dental assistant.
Prerequisites: All required first-semester Dental Assisting courses. Corequisites: All required second-semester Dental Assisting courses. S (N)
|
Dental Hygiene |
|
|
|
|
|
-
DH 120 - Dental Radiography I Credit Hours: 1
The technical aspects of dental radiographic imaging are covered. Lectures include an overview of radiation history, radiation safety and the ALARA principle, a discussion of dental films and digital sensors, intra-oral techniques, film exposure factors and image formation, film processing and mounting, basic image interpretation, quality control techniques and the care and maintenance of dental radiographic equipment. Minimum passing grade is “C-“.
Course Outcomes Upon completion of this course, the student will be able to:
- discuss the rationale for taking dental radiographs;
- comprehend basic radiation safety and protection procedures used in dental radiology;
- describe the process of mounting dental films;
- associate basic geometric theories and principles of the four intra-oral techniques used in the acquisition of quality dental radiographs;
- identify errors and artifacts associated with intraoral technique or processing;
- distinguish various types of radiographic film and image receptors utilized in dentistry;
- explain the details of film processing, and the requirements of a functional darkroom; and
- summarize quality assurance measures as they apply to dental radiography.
Corequisites: All required fall semester, first year courses in the Dental Hygiene or Dental Assisting curricula. Concurrent Registration: DH 121 F (N)
Withdrawal from or failure of a required course prevents further progression in the curriculum. General education courses may be taken earlier than indicated but may not be taken later than the prescribed schedule. |
|
|
|
|
|
|
|
-
DH 175 - Oral Health Services III Credit Hours: 3
Practical experience will be gained in applying the dental hygiene process of care during the treatment and management of dental hygiene patients and will include assessment, planning, implementation, self-assessment and critical thinking for the evaluation of patient care. Experiences will include taking and interpreting digital dental radiographs, application of topical fluorides and varnishes, use of topical anesthetic agents, patient education, instrument sharpening, care of removable dental appliances and the application of sealant materials to prevent tooth decay. Practical exercises in the management of medical emergencies will be conducted. Current infection control and patient confidentiality procedures will be followed. Students must recruit patients for treatment. An introduction to Interprofessional Education is included. Minimum passing grade is “C-” plus completion of specific clinical requirements.
Course Outcomes Upon completion of this course, the student will be able to:
- apply principles of professional, legal and ethical conduct, along with effective professional communication skills in all interactions with patients, peers, and other health care professionals;
- apply medicolegal principles to the systematic collection, analysis and accurate recording of general, personal and oral health status of patients;
- compose a planned sequence of care based on the dental hygiene diagnosis by identifying oral conditions, potential problems, etiologic and risk factors, and available treatment modalities;
- analyze assessment data to implement a basic individualized patient education program;
- choose appropriate preventive and therapeutic treatment procedures to promote and maintain oral health;
- evaluate the effectiveness of planned clinical and educational services and modify as necessary;
- apply evidence-based decision-making knowledge when providing dental hygiene services;
- demonstrate skills for comprehensive patient care to include the placement of sealants; and
- exhibit understanding and application of self-assessment and critical thinking.
Prerequisites: All required second semester courses in the Dental Hygiene curriculum must be passed. Corequisites: Current BLS certification. SS (N)
Withdrawal from or failure of a required course prevents further progression in the curriculum. General education courses may be taken earlier than indicated but may not be taken later than the prescribed schedule. |
|
-
DH 180 - Dental Radiography II Credit Hours: 1
This course is a continuation of Dental Radiography I (DH 120). The fundamentals of dental radiography are explored in greater depth. Lectures will cover an overview of radiation history, patient management, special needs patients, clinical judgment and legal considerations for identifying patient needs for radiographic images, radiation physics and biology, and extraoral imaging. Supplemental techniques will be discussed and include extraoral radiography, digital imaging, and CBCT scans. Minimum required passing grade is “C-.”
Course Outcomes Upon completion of this course, the student will be able to:
- delineate events significant to the development of Radiography in Dentistry;
- summarize the importance of patient management skills and patient education for the acquisition of dental images;
- examine legal issues related to dental radiology;
- assess factors identified in patient’s medical/dental history, clinical assessment, and guidelines established by the FDA for prescribing dental radiographs to meet the individual radiographic need of patients;
- describe how basic physics principles are applied to the charactristic of radiation and x-radiation;
- illustrate the production of dental radiation, including the components of the dental x-ray machine and how it functions;
- decribe radiographic image quality and discuss how quantitative factors influence image quality;
- understand radiation biology and the effects of radiation on living tissues;
- discuss procedures and management techniques for use with pediatric and special needs patients; and
- identify auxiliary radiographic techniques and the rationale for using them in dentistry.
Prerequisites: All required first semester courses in the Dental Hygiene curriculum must be passed. Corequisites: All required spring semester, first year courses in the Dental Hygiene curriculum. Concurrent Registration: DH 181 S (N)
Withdrawal from or failure of a required course prevents further progression in the curriculum. General education courses may be taken earlier than indicated but may not be taken later than the prescribed schedule. |
|
-
DH 181 - Dental Radiography Laboratory II Credit Hours: 1
Practical application of the knowledge gained in DH 180 will include infection control, supplemental intraoral techniques and panoramic radiography. Hands-on training in identification of anatomical landmarks, dental caries, calculus and commonly used restorative dental materials as seen on intraoral and panoramic radiographic images. Minimum passing grade is “C-.”
Course Outcomes Upon completion of this course, the student will be able to:
- apply principles of professional, legal and ethical conduct, along with effective professional communication skills in all interactions with patients, peers, and other health care professionals;
- apply Infection Control Protocol to prevent cross contamination of equipment, image receptors and instruments in a clinical environment;
- integrate descriptive terminology as it relates to dental radiographic images;
- identify and describe normal tooth anatomy and supporting structures as viewed on intraoral or panoramic images;
- differentiate abnormalities, artifacts or foreign objects viewed on radiographic images;
- describe and categorize the radiographic appearance of dental caries utilizing the radiographic classification of caries;
- identify and describe the radiographic appearance of commonly used restorative materials;
- recognize periodontal disease as it appears radiographically utilizing AAP criteria;
- distinguish trauma, pulpal and periapical lesions as seen on radiographic images;
- select supplemental intraoral techniques; and
- identify patient preparation and positioning errors, and articulate corrective action necessary to improve image.
Prerequisites: All required first semester courses in the Dental Hygiene curriculum must be passed. Corequisites: All required spring semester, first year courses in the Dental Hygiene curriculum. Concurrent Registration: DH 180 S (N)
Withdrawal from or failure of a required course prevents further progression in the curriculum. General education courses may be taken earlier than indicated but may not be taken later than the prescribed schedule. |
|
|
|
Page: 1
| 2
| 3
| 4
| 5
| 6
| 7
| 8
| 9
| 10
| 11
-> 14 |
|
|